Witam!
Chcę stworzyć aplikację Winforms która będzie uwierzytelniala się do pewnego API poprzez OAuth 2. Jak na razie działą to tak, że na serwerze jest plik *.php który to akceptuje zapytanie z tokenem. Ale wiem, że niektóre aplikacje mają to rozwiązane na zasadzie jakiegoś callbacku czy jak to tam się nazywa.
Szukałem w Internecie i niestety niczego nie znalazłem. Czy ktoś mógłby mi powiedzieć co mam zrobić?
- Rejestracja:ponad 6 lat
- Ostatnio:prawie 5 lat
- Postów:43
- Rejestracja:około 22 lata
- Ostatnio:około miesiąc
- Postów:5042
To jakoś kiepsko szukałeś albo nie rozumiem dokładnie z czym masz problem:
https://oauth.net/2/
https://sekurak.pl/oauth-2-0-jak-dziala-jak-testowac-problemy-bezpieczenstwa/
https://docs.microsoft.com/pl-pl/azure/active-directory/develop/v1-protocols-oauth-code
Na pewno też znajdziesz na NuGet coś do OAuth2. Wiele lat temu pisałem na własne potrzeby, ale na pewno coś znajdziesz gotowego.
- Rejestracja:ponad 5 lat
- Ostatnio:około 2 lata
- Postów:105
@Juhas: ale żeś kolegę zawalił teorią ;-)
@Gushasad praktyce to robisz coś takiego:
https://www.codeproject.com/Tips/359144/2-Legged-OAuth-Authentication-in-NET-Csharp
W jednym z projektów używam Google APIs Client Library for .NET, kod robiący Oauth możesz sobie tu poczytać i odpowiednio dostosować:
https://github.com/googleapis/google-api-dotnet-client/tree/master/Src/Generated/Google.Apis.Oauth2.v2
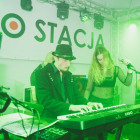
- Rejestracja:ponad 13 lat
- Ostatnio:około 5 lat
- Postów:839
Kiedy uczyłem się pobierać i wysyłąć dane poprzez RestAPI stworzyłem coś takiego:
/// <summary>
/// Interfejs wykorzystywany przez metodę <see cref="MuzoStacjaApi.POST(string, ISerialize)" do generowania ciągu JSON/>
/// </summary>
public interface ISerialize
{
/// <summary>
/// Zwraca klasę mającą takie same nazwy właściwości jak te występujące w ciągu JSON
/// </summary>
/// <returns></returns>
object Get();
}
public abstract class SerializedClass
{
public class Default : ISerialize
{
public object Get()
{
return this;
}
}
/// <summary>
/// Klasa stworzona potrzeby utworzenia ciągu JSON potrzebnego do uzyskania Tokenu sesji
/// </summary>
public class TokenCredential : ISerialize
{
/// <summary>
/// Tworzy nową instację obiektu
/// </summary>
/// <param name="Email">Email lub numer telefonu</param>
/// <param name="Password">Hasło użytkownika</param>
public TokenCredential(string Email, string Password)
{
customer = new Customer(Email, Password);
}
public class Customer
{
public Customer(string Email, string Password)
{
email = Email;
password = Password;
}
public string email { get; private set; }
public string password { get; private set; }
}
public Customer customer { get; private set; }
public object Get()
{
return this;
}
}
}
public static class Plan4uApi
{
public static PostResult POST(string Address, string Arguments, ISerialize Body)
{
PostResult OperationResult = null;
try
{
if (string.IsNullOrEmpty(Address))
throw new Exception("Pole Address nie może być puste");
if (Address[Address.Count() - 1] != '/')
Address += "/";
Address += Arguments;
ServicePointManager.Expect100Continue = true;
ServicePointManager.SecurityProtocol = SecurityProtocolType.Tls12;
var httpWebRequest = (HttpWebRequest)WebRequest.Create(Address);
httpWebRequest.ContentType = "application/json";
httpWebRequest.Method = "POST";
using (var streamWriter = new StreamWriter(httpWebRequest.GetRequestStream()))
{
if (Body != null)
{
string json = JsonConvert.SerializeObject(Body.Get());
streamWriter.Write(json);
}
}
var httpResponse = (HttpWebResponse)httpWebRequest.GetResponse();
using (var streamReader = new StreamReader(httpResponse.GetResponseStream()))
{
string result = streamReader.ReadToEnd();
return new PostResult(result);
}
}
catch (WebException WebException)
{
OperationResult = new PostResult(ePostResult.WebException, WebException);
}
catch (ObjectDisposedException ObjectDisposedException)
{
OperationResult = new PostResult(ePostResult.ObjectDisposedException, ObjectDisposedException);
}
catch (NotSupportedException NotSupportedException)
{
OperationResult = new PostResult(ePostResult.NotSupportedException, NotSupportedException);
}
catch (ArgumentNullException ArgumentNullException)
{
OperationResult = new PostResult(ePostResult.ArgumentNullException, ArgumentNullException);
}
catch (System.Security.SecurityException SecurityException)
{
OperationResult = new PostResult(ePostResult.SecurityException, SecurityException);
}
catch (UriFormatException UriFormatException)
{
OperationResult = new PostResult(ePostResult.UriFormatException, UriFormatException);
}
catch (ArgumentException ArgumentException)
{
OperationResult = new PostResult(ePostResult.ArgumentException, ArgumentException);
}
catch (ProtocolViolationException ProtocolViolationException)
{
OperationResult = new PostResult(ePostResult.ProtocolViolationException, ProtocolViolationException);
}
catch (InvalidOperationException InvalidOperationException)
{
OperationResult = new PostResult(ePostResult.InvalidOperationException, InvalidOperationException);
}
catch (OutOfMemoryException OutOfMemoryException)
{
OperationResult = new PostResult(ePostResult.OutOfMemoryException, OutOfMemoryException);
}
catch (IOException IOException)
{
OperationResult = new PostResult(ePostResult.IOException, IOException);
}
catch (Exception Exception)
{
OperationResult = new PostResult(ePostResult.Exception, Exception);
}
return OperationResult;
}
public static GetResult GET(string Address, string Arguments, ResponseToken TokenData)
{
GetResult OperationResult = null;
try
{
if (string.IsNullOrEmpty(Address))
throw new Exception("Pole Address nie może być puste");
if (Address[Address.Count() - 1] != '/')
Address += "/";
Address += Arguments;
ServicePointManager.Expect100Continue = true;
ServicePointManager.SecurityProtocol = SecurityProtocolType.Tls12;
var httpWebRequest = (HttpWebRequest)WebRequest.Create(Address);
//httpWebRequest.ContentType = "application/json";
httpWebRequest.Method = "GET";
if (!TokenData.TokenExist)
throw new InvalidTokenException("Niepoprawny Token sesji");
httpWebRequest.Headers.Add(HttpRequestHeader.Authorization, TokenData.Token);
var httpResponse = (HttpWebResponse)httpWebRequest.GetResponse();
using (var streamReader = new StreamReader(httpResponse.GetResponseStream()))
{
string result = streamReader.ReadToEnd();
return new GetResult(result);
}
}
catch (WebException WebException)
{
OperationResult = new GetResult(eGetResult.WebException, WebException);
}
catch (ObjectDisposedException ObjectDisposedException)
{
OperationResult = new GetResult(eGetResult.ObjectDisposedException, ObjectDisposedException);
}
catch (NotSupportedException NotSupportedException)
{
OperationResult = new GetResult(eGetResult.NotSupportedException, NotSupportedException);
}
catch (ArgumentNullException ArgumentNullException)
{
OperationResult = new GetResult(eGetResult.ArgumentNullException, ArgumentNullException);
}
catch (System.Security.SecurityException SecurityException)
{
OperationResult = new GetResult(eGetResult.SecurityException, SecurityException);
}
catch (UriFormatException UriFormatException)
{
OperationResult = new GetResult(eGetResult.UriFormatException, UriFormatException);
}
catch (ArgumentException ArgumentException)
{
OperationResult = new GetResult(eGetResult.ArgumentException, ArgumentException);
}
catch (ProtocolViolationException ProtocolViolationException)
{
OperationResult = new GetResult(eGetResult.ProtocolViolationException, ProtocolViolationException);
}
catch (InvalidOperationException InvalidOperationException)
{
OperationResult = new GetResult(eGetResult.InvalidOperationException, InvalidOperationException);
}
catch (OutOfMemoryException OutOfMemoryException)
{
OperationResult = new GetResult(eGetResult.OutOfMemoryException, OutOfMemoryException);
}
catch (IOException IOException)
{
OperationResult = new GetResult(eGetResult.IOException, IOException);
}
catch (InvalidTokenException InvalidTokenException)
{
OperationResult = new GetResult(eGetResult.InvalidTokenException, InvalidTokenException);
}
catch (Exception Exception)
{
OperationResult = new GetResult(eGetResult.Exception, Exception);
}
return OperationResult;
}
}
Nie wstawiłem całego kodu, ogólnie potrzebowałem wywołać POST aby pobrać token sesji za pomocą loginu i hasła, które przesłałem w BODY. Potem już tylko GET dla pobierania danych. Czy to jakoś pomogło?