@DibbyDum, @teo215 Czy chodziło o taki serwis?
using System;
using System.Collections.Generic;
using System.Collections.ObjectModel;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Library.Model;
namespace Library.Services {
public interface ILibraryService {
ICollection<Book> GetBooks();
ICollection<Author> GetAuthors();
ICollection<BooksView> GetBooksView();
bool InsertBook(Book book);
bool InsertAuthor(Author author);
bool Associate(int bookId, int authorId);
}
public class LibraryService : ILibraryService {
public ICollection<Book> GetBooks() {
using (var db = new LibraryContext()) {
return db.Books.ToList();
}
}
public ICollection<Author> GetAuthors() {
using (var db = new LibraryContext()) {
return db.Authors.ToList();
}
}
public ICollection<BooksView> GetBooksView() {
using (var db = new LibraryContext()) {
return db.BooksView.ToList();
}
}
public bool InsertBook(Book book) {
try {
using (var db = new LibraryContext()) {
db.Books.Add(book);
return db.SaveChanges() > 0;
}
}
catch (Exception) {
return false;
}
}
public bool InsertAuthor(Author author) {
try {
using (var db = new LibraryContext()) {
db.Authors.Add(author);
return db.SaveChanges() > 0;
}
}
catch (Exception) {
return false;
}
}
public bool Associate(int bookId, int authorId) {
try {
using (var db = new LibraryContext()) {
var book = db.Books.Single(b => b.Id == bookId);
var author = db.Authors.Single(a => a.Id == authorId);
book.Authors.Add(author);
author.Books.Add(book);
return db.SaveChanges() > 0;
}
}
catch (Exception ex) {
System.Windows.MessageBox.Show(ex.ToString());
return false;
}
}
}
}
Generalnie baza jest prosta. Taka tam... żeby ogarnąć działanie ORM:
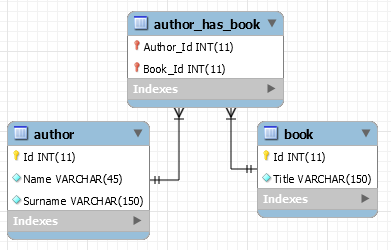
Klasy Book
i Author
to fizyczne tabele w bazie danych. Klasa BookView
to klasa, która bierze dane z widoku zrobionego w bazie.
Czy chodziło o takie (albo podobne) podejście? :)
PS: Nie zwracajcie uwagi na ogolny Exception
i to, że jest tam tylko return false
. Chodzi generalnie o ideę serwisu. W viewmodelu będzie luźna referencja w postaci interfejsu.